Astroでプロジェクトを始めたものの、ファイルやフォルダをどう整理すれば良いか悩んでいませんか?
初期構成のフォルダの役割がイマイチ分からず、大規模になったときの管理に不安を感じていませんか?
この記事では、Astroのディレクトリ構成を初心者でも理解できるよう、実践的な例とコード付きで詳しく解説します。
将来の拡張性と保守性を見据えたプロジェクト設計のノウハウをご紹介します。
- この記事を読んでわかること
- Astroの基本ディレクトリ構成(src/pages, src/components, src/layouts)の意味と役割
- publicフォルダとsrc/assetsの適切な使い分け方
- コンポーネントの粒度に応じた効率的な配置方法
- 複数のサブディレクトリを使ったカテゴリ分けの実装テクニック
- MarkdownやMDXファイルを活用したブログ構築のベストプラクティス
- Astro.glob()やimport.meta.glob()を使った自動読み込みの実装方法
- 複数人開発でも混乱しない命名規則と構成ルール
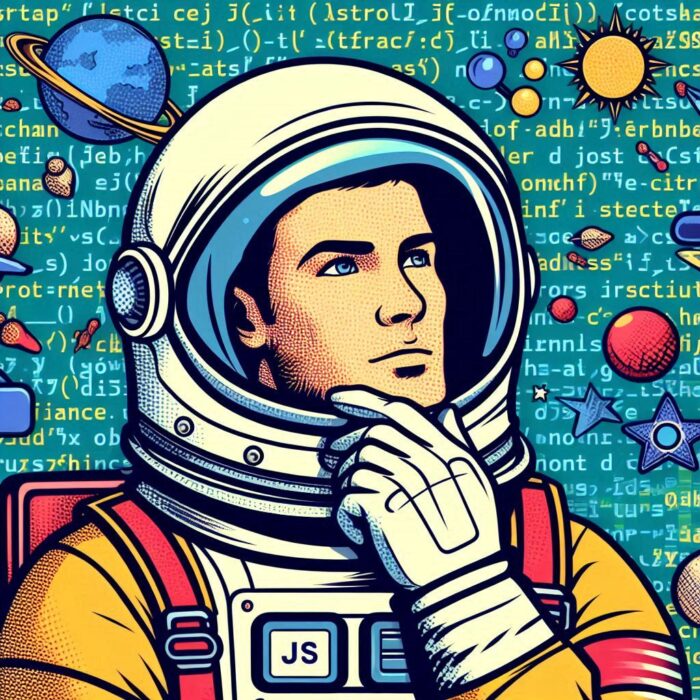
Astroとは?
Astroは、HTMLファーストで設計された次世代のWebフレームワークです。React/Vue/SvelteなどのUIライブラリを統合しつつ、必要な箇所だけJavaScriptを読み込む「アイランドアーキテクチャ」が特徴です。特に静的サイトの構築に強みを持ち、高速なWebサイト作成を可能にします。
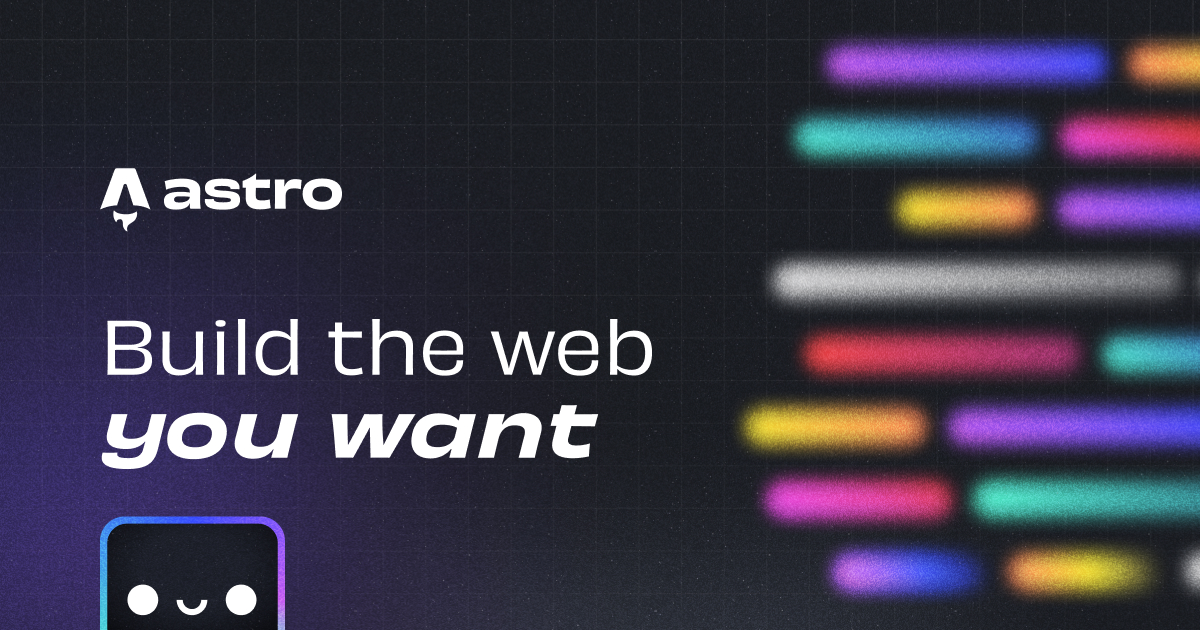
Astroの基本ディレクトリ構成とその役割
Astroプロジェクトを初めて作成したとき、下記のようなディレクトリ構造が生成されます。まずはこの基本構造を理解することが、効率的な開発の第一歩です。
my-astro-project/
├─ src/
│ ├─ components/
│ ├─ layouts/
│ ├─ pages/
│ ├─ styles/
│ └─ assets/
├─ public/
├─ astro.config.mjs
├─ package.json
└─ tsconfig.json
これらのディレクトリは、単なる分類以上の意味を持っています。それぞれが特定の役割を担い、Astroの動作に直接影響を与えます。実家の間取りに例えるなら、リビングや寝室などの部屋がそれぞれ特定の目的を持つように、Astroのディレクトリも明確な役割分担があるのです。
src/pages, src/components, src/layouts の違いと使い分け
src/pages
このディレクトリは、ウェブサイトのページルーティングを直接制御します。ここに置いたファイルがそのままURLのパスになる「ファイルベースルーティング」の仕組みです。
例えば:
src/pages/index.astro
→yourdomain.com/
(ホームページ)src/pages/about.astro
→yourdomain.com/about
(アバウトページ)src/pages/blog/post-1.md
→yourdomain.com/blog/post-1
(ブログ記事)
これはNext.jsなどでも採用されている直感的な仕組みで、URLとファイル構造が完全に一致するため、サイト構造が視覚的に理解しやすくなります。
また、この特性を活かして、動的ルーティングも実現できます:
---
// src/pages/blog/[slug].astro
export async function getStaticPaths() {
const posts = await Astro.glob('../data/posts/*.md');
return posts.map(post => ({
params: { slug: post.frontmatter.slug },
props: { post }
}));
}
const { post } = Astro.props;
---
<h1>{post.frontmatter.title}</h1>
<article set:html={post.compiledContent()} />
src/components
コンポーネントは、再利用可能なUIの部品です。ボタン、ナビゲーション、カード、フォームなど、サイト全体で繰り返し使用される要素をここに配置します。
Astroのコンポーネントは.astro
拡張子を持ち、HTMLのようなシンタックスとJavaScriptのフロントマターを組み合わせた構造になっています:
---
// src/components/Button.astro
const { text, color = "blue" } = Astro.props;
---
<button class={`btn btn-${color}`}>
{text}
</button>
<style>
.btn {
padding: 0.5rem 1rem;
border-radius: 0.25rem;
}
.btn-blue {
background-color: #3182ce;
color: white;
}
.btn-green {
background-color: #38a169;
color: white;
}
</style>
このコンポーネントはページで次のように使用できます:
---
// src/pages/index.astro
import Button from '../components/Button.astro';
---
<Button text="送信する" color="green" />
src/layouts
レイアウトは、複数ページで共有されるページ構造を定義するための特別なコンポーネントです。ヘッダー、フッター、サイドバーなど、サイト全体で一貫した要素を含みます。
技術的には通常のコンポーネントと同じですが、用途と慣習として分けられています。典型的なレイアウトは以下のようになります:
---
// src/layouts/MainLayout.astro
import Header from '../components/Header.astro';
import Footer from '../components/Footer.astro';
const { title } = Astro.props;
---
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>{title} | My Astroサイト</title>
</head>
<body>
<Header />
<main>
<slot /> <!-- ここにページコンテンツが挿入されます -->
</main>
<Footer />
</body>
</html>
ページ内では、以下のように使用します:
---
// src/pages/about.astro
import MainLayout from '../layouts/MainLayout.astro';
---
<MainLayout title="About Us">
<h1>私たちについて</h1>
<p>会社の沿革や理念をここに記載します。</p>
</MainLayout>
コンポーネント(src/components
)とレイアウト(src/layouts
)の主な違いは「用途」です。コンポーネントはボタンやカードなどの小さな部品に、レイアウトはページ全体の枠組みに使用されます。例えるなら、コンポーネントは家具や家電、レイアウトは家の間取りや壁のような役割です。
publicとsrc/assetsの画像管理のベストプラクティス
Astroでは、画像や静的ファイルを管理するための2つの主要なディレクトリがあります:public
とsrc/assets
です。両者の違いを理解することで、効率的な画像最適化が可能になります。
public ディレクトリ
public
ディレクトリに配置したファイルは、一切処理されずにそのまま出力ディレクトリにコピーされます。つまり:
- 最適化されない
- ファイル名が変更されない
- URLパスが完全に予測可能
これは以下のような場合に適しています:
- ファビコン
- robots.txt
- サイトマップ
- フォントファイル
- すでに最適化済みの画像
- 動的に生成されたイメージのパスとして使用される画像
使用例:
<img src="/images/logo.png" alt="ロゴ画像" />
src/assets ディレクトリ
Astro 2.0以降で導入されたsrc/assets
ディレクトリは、自動的に最適化される画像を格納するために使用されます。以下のようなメリットがあります:
- 画像の圧縮
- 複数のフォーマット(WebP、AVIFなど)の生成
- 複数の解像度(Responsive Images)の生成
- コンテンツハッシュによるファイル名変更(キャッシュバスティング)
使用例:
---
import { Image } from 'astro:assets';
import myImage from '../assets/images/photo.jpg';
---
<Image src={myImage} alt="最適化された写真" width={800} height={600} />
使い分けのベストプラクティス
実際のプロジェクトでは、以下のような使い分けが効果的です:
Astroの初期構成を理解する:最小構成とその意味
Astroプロジェクトの最小構成を理解することで、その設計思想がより明確になります。Astroの基本哲学は「シンプルなことをシンプルに保つ」ということ。必要なものだけを含める設計思想が、初期構成にも表れています。
最小限のAstroプロジェクトは、実は以下の3つのファイルだけで構成できます:
my-minimal-astro/
├─ src/
│ └─ pages/
│ └─ index.astro
├─ astro.config.mjs
└─ package.json
これだけでも完全に機能するウェブサイトをビルドできる点が、Astroの強みです。必要に応じて段階的に機能を追加していくアプローチが可能です。
たとえば、最小限のindex.astro
は以下のようになります:
---
// src/pages/index.astro
---
<html lang="ja">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My Minimal Astro Site</title>
</head>
<body>
<h1>Hello, Astro!</h1>
</body>
</html>
そして、astro.config.mjs
も以下のように最小限に保つことができます:
// astro.config.mjs
import { defineConfig } from 'astro/config';
export default defineConfig({});
この最小構成から出発し、必要に応じて機能を追加していくことで、不要な複雑さを避けつつ、プロジェクトに最適な構造を構築できます。これは「YAGNI(You Aren’t Gonna Need It:必要になるまで作らない)」原則に基づくアプローチであり、無駄な開発リソースを削減します。

実践的なディレクトリ構成の設計ガイド
小さなプロジェクトであれば、初期構成のままでも問題なく開発を進められます。しかし、サイトの規模が大きくなるにつれて、ファイル数が増加し、管理が難しくなってきます。まるで整理整頓されていない引き出しのように、必要なものを探すのに時間がかかってしまうのです。
そこで、実践的なディレクトリ構成の設計について、具体的な例を交えながら解説していきます。
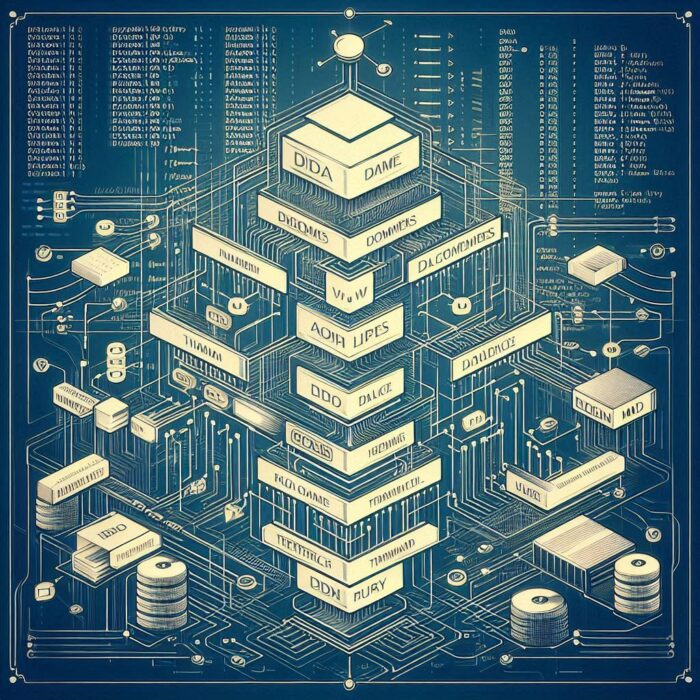
コンポーネント粒度の目安と配置例(Header, Footer, Button など)
コンポーネントの粒度は、プロジェクトの規模や開発チームの方針によって異なりますが、一般的には以下のような分類が効果的です。
粒度による分類の例
src/
components/
ui/ # 最小単位のUIコンポーネント
Button.astro
Input.astro
Card.astro
blocks/ # 複数のUIコンポーネントで構成される中規模コンポーネント
SearchForm.astro
PricingCard.astro
sections/ # ページの主要セクションを構成する大規模コンポーネント
Hero.astro
Features.astro
global/ # 複数ページで共通して使用されるコンポーネント
Header.astro
Footer.astro
Navigation.astro
この構成では、コンポーネントを「粒度」と「用途」によって整理しています。例えば、Button.astro
のような再利用性の高い小さなコンポーネントはui
ディレクトリに、Header.astro
のような全ページ共通のコンポーネントはglobal
ディレクトリに配置します。
コンポーネント粒度の目安
実際のコードで見てみましょう。例えば、Button.astroは次のように実装できます:
---
// src/components/ui/Button.astro
export interface Props {
variant?: 'primary' | 'secondary' | 'outline';
size?: 'sm' | 'md' | 'lg';
href?: string;
}
const {
variant = 'primary',
size = 'md',
href
} = Astro.props;
const getVariantClasses = () => {
switch(variant) {
case 'primary':
return 'bg-blue-600 text-white hover:bg-blue-700';
case 'secondary':
return 'bg-gray-200 text-gray-800 hover:bg-gray-300';
case 'outline':
return 'border border-blue-600 text-blue-600 hover:bg-blue-50';
default:
return 'bg-blue-600 text-white hover:bg-blue-700';
}
};
const getSizeClasses = () => {
switch(size) {
case 'sm':
return 'text-sm py-1 px-2';
case 'md':
return 'text-base py-2 px-4';
case 'lg':
return 'text-lg py-3 px-6';
default:
return 'text-base py-2 px-4';
}
};
const classes = `
${getVariantClasses()}
${getSizeClasses()}
rounded font-medium transition-colors
`;
---
{
href ? (
<a href={href} class={classes}>
<slot />
</a>
) : (
<button class={classes}>
<slot />
</button>
)
}
そして、このButtonコンポーネントを使用した、より大きなHeroセクションコンポーネントは次のようになります:
---
// src/components/sections/Hero.astro
import Button from '../ui/Button.astro';
---
<section class="py-20 bg-gradient-to-r from-blue-500 to-purple-600 text-white">
<div class="container mx-auto px-4">
<div class="max-w-2xl">
<h1 class="text-4xl md:text-5xl font-bold mb-6">
<slot name="title">デフォルトタイトル</slot>
</h1>
<p class="text-xl mb-8 opacity-90">
<slot name="description">デフォルト説明テキスト</slot>
</p>
<div class="flex flex-wrap gap-4">
<Button variant="primary" size="lg">
<slot name="primary-cta">始める</slot>
</Button>
<Button variant="outline" size="lg">
<slot name="secondary-cta">詳細を見る</slot>
</Button>
</div>
</div>
</div>
</section>
コンポーネントの粒度を適切に設計することで、コードの再利用性が高まり、メンテナンス性も向上します。例えば、サイト全体のボタンスタイルを変更したい場合、Button.astro
ファイル1つを修正するだけで済みます。
複数のサブディレクトリでカテゴリ分けする方法(例:/blog/, /works/)
Astroでは、src/pages
ディレクトリの構造がそのままURLパスに反映されます。これを活用して、コンテンツをカテゴリごとに整理することができます。
基本的なサブディレクトリ構成
src/
pages/
index.astro # -> example.com/
about.astro # -> example.com/about
contact.astro # -> example.com/contact
blog/ # ブログ関連ページ
index.astro # -> example.com/blog/
[slug].astro # -> example.com/blog/{slug}
works/ # 制作実績関連ページ
index.astro # -> example.com/works/
[id].astro # -> example.com/works/{id}
このようにディレクトリを分けることで、URLの階層構造が明確になり、SEOにも有利です。また、コンテンツタイプごとに異なるレイアウトやロジックを適用しやすくなります。
動的ルーティングを活用した例
例えば、ブログ記事一覧ページと個別記事ページを実装する場合:
---
// src/pages/blog/index.astro
import Layout from '../../layouts/BlogLayout.astro';
import { getCollection } from 'astro:content';
const posts = await getCollection('blog');
---
<Layout title="ブログ記事一覧">
<h1>ブログ記事一覧</h1>
<ul>
{posts.map(post => (
<li>
<a href={`/blog/${post.slug}`}>
{post.data.title}
<time datetime={post.data.publishDate.toISOString()}>
{post.data.publishDate.toLocaleDateString('ja-JP')}
</time>
</a>
</li>
))}
</ul>
</Layout>
そして、動的ルーティングを使用した個別記事ページ:
---
// src/pages/blog/[slug].astro
import { getCollection } from 'astro:content';
import Layout from '../../layouts/BlogPostLayout.astro';
export async function getStaticPaths() {
const blogEntries = await getCollection('blog');
return blogEntries.map(entry => ({
params: { slug: entry.slug },
props: { entry },
}));
}
const { entry } = Astro.props;
const { Content } = await entry.render();
---
<Layout title={entry.data.title}>
<article>
<h1>{entry.data.title}</h1>
<time datetime={entry.data.publishDate.toISOString()}>
{entry.data.publishDate.toLocaleDateString('ja-JP')}
</time>
<Content />
</article>
</Layout>
この方法で、/blog/astro-directory-structure
のようなURLが自動的に生成されます。同様に、制作実績やサービス紹介など、他のコンテンツタイプにも適用できます。
言語別ディレクトリの例
多言語サイトを構築する場合は、言語ごとにサブディレクトリを設けるアプローチも効果的です:
src/
pages/
index.astro # デフォルト言語(日本語)
en/ # 英語
index.astro
about.astro
fr/ # フランス語
index.astro
about.astro
このアプローチでは、各言語ごとに専用のコンテンツファイルを用意するため、翻訳管理が直感的になります。
ブログ向け:Markdown・MDXファイルの配置と構造パターン
ブログやドキュメントサイトでは、多くの場合、Markdownファイルを使ってコンテンツを管理します。Astroでは、コンテンツコレクション機能を使用することで、効率的にMarkdownファイルを管理できます。
コンテンツコレクションを使用したパターン
まず、src/content
ディレクトリにコレクションを定義します:
src/
content/
config.ts # コレクション定義ファイル
blog/ # ブログ記事用コレクション
post-1.md
post-2.md
post-3.mdx
authors/ # 著者情報用コレクション
john-doe.json
jane-smith.json
config.ts
には、各コレクションのスキーマを定義します:
// src/content/config.ts
import { defineCollection, z } from 'astro:content';
const blogCollection = defineCollection({
schema: z.object({
title: z.string(),
description: z.string(),
publishDate: z.date(),
author: z.string(),
image: z.string().optional(),
tags: z.array(z.string()).default([]),
}),
});
const authorsCollection = defineCollection({
schema: z.object({
name: z.string(),
bio: z.string(),
avatar: z.string(),
socialLinks: z.record(z.string()).optional(),
}),
});
export const collections = {
'blog': blogCollection,
'authors': authorsCollection,
};
そして、ブログ記事のMarkdownファイルは以下のようになります:
---
title: "Astroでの効率的なディレクトリ構成について"
description: "Astroプロジェクトを整理するためのベストプラクティスを紹介します"
publishDate: 2023-05-15
author: "john-doe"
image: "/images/blog/directory-structure.jpg"
tags: ["Astro", "Web開発", "ディレクトリ構成"]
---
# Astroでの効率的なディレクトリ構成について
ここにマークダウンコンテンツが入ります...
これらのコンテンツを取得して表示する例:
---
// src/pages/blog/index.astro
import { getCollection } from 'astro:content';
import Layout from '../../layouts/BlogLayout.astro';
const posts = await getCollection('blog');
const sortedPosts = posts.sort(
(a, b) => b.data.publishDate.getTime() - a.data.publishDate.getTime()
);
---
<Layout title="ブログ">
<h1>最新の記事</h1>
<div class="post-grid">
{sortedPosts.map(post => (
<article class="post-card">
{post.data.image && (
<img
src={post.data.image}
alt={post.data.title}
loading="lazy"
/>
)}
<h2><a href={`/blog/${post.slug}`}>{post.data.title}</a></h2>
<p>{post.data.description}</p>
<div class="post-meta">
<time datetime={post.data.publishDate.toISOString()}>
{post.data.publishDate.toLocaleDateString('ja-JP')}
</time>
</div>
</article>
))}
</div>
</Layout>
カテゴリ別やシリーズ別の構造
ブログ記事を「カテゴリ」や「シリーズ」で分類したい場合は、次のような構造も検討できます:
src/
content/
blog/
javascript/ # JavaScriptカテゴリ
basics.md
advanced.md
astro/ # Astroカテゴリ
getting-started.md
directory-structure.md
この場合、スラグはjavascript/basics
のような形式になります。これを利用して、カテゴリ別のアーカイブページを作成することも可能です:
---
// src/pages/blog/category/[category].astro
import { getCollection } from 'astro:content';
import Layout from '../../../layouts/BlogLayout.astro';
export async function getStaticPaths() {
const posts = await getCollection('blog');
// スラグからカテゴリを抽出(最初の/までの部分)
const categories = [...new Set(
posts.map(post => {
const parts = post.slug.split('/');
return parts.length > 1 ? parts[0] : 'uncategorized';
})
)];
return categories.map(category => ({
params: { category },
props: {
posts: posts.filter(post => post.slug.startsWith(category + '/'))
},
}));
}
const { category } = Astro.params;
const { posts } = Astro.props;
---
<Layout title={`${category}カテゴリの記事`}>
<h1>{category}カテゴリの記事</h1>
<ul>
{posts.map(post => (
<li>
<a href={`/blog/${post.slug}`}>{post.data.title}</a>
</li>
))}
</ul>
</Layout>
このように、コンテンツコレクション機能を活用することで、大量のMarkdownファイルも効率的に管理できます。特に、タイプセーフなスキーマ定義により、フロントマターの入力ミスを防ぐことができるため、複数人での開発や長期運用に適しています。
実際のプロジェクトでは、これらのパターンを組み合わせて、コンテンツの特性に合わせた最適な構造を設計することをおすすめします。例えば、シンプルなブログであれば平坦な構造で十分ですが、複雑な製品ドキュメントサイトであれば、階層構造を採用する方が管理しやすいでしょう。
ディレクトリ構造は、プロジェクトの「地図」のようなものです。適切に設計されていれば、新しいメンバーも迷わずに開発に参加でき、既存メンバーも効率的に作業を進められます。ぜひ、プロジェクトの特性や開発チームの規模に合わせて、最適な構造を検討してみてください。

拡張性・保守性を高めるための上級ディレクトリ設計
プロジェクトが成長するにつれて、単にファイルを適切な場所に配置するだけでなく、将来の拡張や保守を見据えた高度なディレクトリ設計が重要になってきます。これは、長期的なプロジェクトにおいて「技術的負債」を減らし、開発効率を維持するための重要な投資です。
ここでは、Astroプロジェクトの拡張性と保守性を高めるための上級テクニックを紹介します。
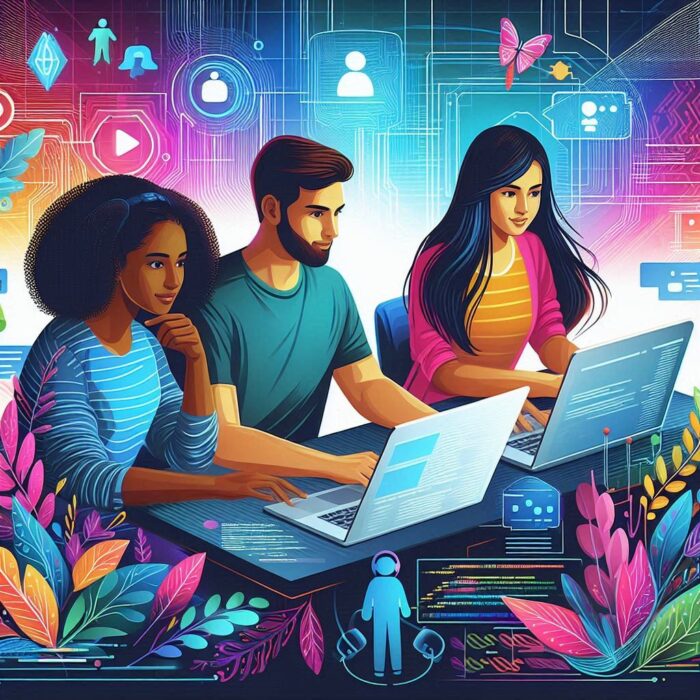
Astro.glob()とimport.meta.glob()を活用した自動読み込みと構造設計
Astroは、ファイルシステムに基づいたルーティングだけでなく、ファイルの自動読み込み機能も提供しています。これらを活用することで、手動でのインポート管理を減らし、拡張性の高い設計が可能になります。
Astro.glob()の基本的な使い方
Astro.glob()
は、指定したパターンに一致するすべてのファイルを一度にインポートするための関数です。例えば、すべてのブログ記事を取得する場合:
---
// すべてのMarkdownファイルを取得
const allPosts = await Astro.glob('../content/blog/**/*.md');
// 公開日でソート
const sortedPosts = allPosts
.filter(post => !post.frontmatter.draft)
.sort((a, b) =>
new Date(b.frontmatter.publishDate).getTime() -
new Date(a.frontmatter.publishDate).getTime()
);
---
<ul>
{sortedPosts.map(post => (
<li>
<a href={post.url}>{post.frontmatter.title}</a>
<time datetime={post.frontmatter.publishDate}>
{new Date(post.frontmatter.publishDate).toLocaleDateString('ja-JP')}
</time>
</li>
))}
</ul>
ただし、コンテンツコレクションが利用可能な場合は、そちらの使用が推奨されています。
import.meta.glob()を使った動的インポート
より高度な使い方として、import.meta.glob()
を使用すると、Viteの機能を活用して動的インポートができます。これはコンポーネントやモジュールの自動読み込みに特に便利です:
---
// すべてのコンポーネントを動的にインポート
const components = import.meta.glob('../components/ui/*.astro');
// 使用するコンポーネント名
const componentName = 'Button';
// パスを構築
const componentPath = `../components/ui/${componentName}.astro`;
// コンポーネントが存在するか確認
const Component = components[componentPath]
? (await components[componentPath]()).default
: null;
---
{Component && <Component />}
この手法を利用すると、プラグインのような拡張性の高いシステムを構築できます。例えば、特定のディレクトリに配置されたすべてのブロックコンポーネントを自動的に読み込み、ブロックライブラリとして提供することができます:
---
// src/pages/block-library.astro
// すべてのブロックコンポーネントを取得
const blockModules = import.meta.glob('../components/blocks/*.astro');
const blocks = Object.keys(blockModules).map(path => {
// ファイル名(拡張子なし)を取得
const name = path.split('/').pop().replace('.astro', '');
return { name, path };
});
// 各ブロックを動的にレンダリングする関数
async function renderBlock(block) {
const module = await blockModules[block.path]();
const Block = module.default;
return Block;
}
---
<html lang="ja">
<head>
<title>ブロックライブラリ</title>
<style>
.block-preview {
margin: 2rem 0;
padding: 1rem;
border: 1px solid #ddd;
border-radius: 0.5rem;
}
</style>
</head>
<body>
<h1>利用可能なブロックコンポーネント</h1>
{blocks.map(async (block) => {
const Block = await renderBlock(block);
return (
<div class="block-preview">
<h2>{block.name}</h2>
<Block />
</div>
);
})}
</body>
</html>
自動読み込みを活用したディレクトリ構造の例
この機能を活かしたディレクトリ構造の例を見てみましょう:
src/
components/
blocks/ # 自動読み込み対象のブロックコンポーネント
Hero.astro
Features.astro
Testimonials.astro
ui/ # 基本UIコンポーネント
Button.astro
Card.astro
layouts/
BaseLayout.astro # 基本レイアウト
pages/
index.astro
block-library.astro # ブロックライブラリページ
utils/
components.js # コンポーネント読み込みユーティリティ
そして、components.js
には以下のようなユーティリティ関数を定義します:
// src/utils/components.js
// 指定したディレクトリのコンポーネントをすべて読み込む
export function loadComponents(directory) {
return import.meta.glob(`../components/${directory}/*.astro`);
}
// コンポーネントを名前で動的に読み込む
export async function getComponentByName(directory, name) {
const components = loadComponents(directory);
const path = `../components/${directory}/${name}.astro`;
if (components[path]) {
const module = await components[path]();
return module.default;
}
return null;
}
このユーティリティを使用すると、例えばCMSから取得したデータに基づいて動的にコンポーネントをレンダリングできます:
---
// src/pages/dynamic-page.astro
import { getComponentByName } from '../utils/components';
// CMSから取得したデータを想定
const pageData = {
sections: [
{ type: 'Hero', props: { title: 'メインタイトル', subtitle: 'サブタイトル' } },
{ type: 'Features', props: { features: [/*...*/] } },
{ type: 'Testimonials', props: { testimonials: [/*...*/] } }
]
};
// 各セクションのコンポーネントを取得
const sectionComponents = await Promise.all(
pageData.sections.map(async section => {
const Component = await getComponentByName('blocks', section.type);
return { Component, props: section.props };
})
);
---
<html lang="ja">
<head>
<title>動的ページ</title>
</head>
<body>
{sectionComponents.map(({ Component, props }) =>
Component ? <Component {...props} /> : null
)}
</body>
</html>
この設計により、新しいブロックコンポーネントを追加するだけで、CMSから制御可能なページを容易に拡張できます。
ルーティングとファイルパスの関係を正しく設計する方法
Astroのファイルベースルーティングは直感的ですが、大規模プロジェクトでは、より詳細な設計が必要です。ここでは、いくつかの高度なルーティングパターンを紹介します。
パラメータを活用した動的ルーティング
基本的な動的ルーティングは[param].astro
で実現できますが、複数のパラメータや任意のパラメータを組み合わせることで、より柔軟なルーティングが可能になります:
src/
pages/
products/
[category]/
index.astro # /products/category名/
[productId].astro # /products/category名/product-id
[...slug].astro # その他のすべてのパス(404対応)
例えば、[category]/[productId].astro
は次のように実装できます:
---
// src/pages/products/[category]/[productId].astro
import ProductLayout from '../../../layouts/ProductLayout.astro';
import { getProductByIdAndCategory } from '../../../data/products';
export async function getStaticPaths() {
// すべての商品データを取得(例)
const allProducts = [
{ id: 'product-1', category: 'electronics', name: 'スマートフォン' },
{ id: 'product-2', category: 'electronics', name: 'タブレット' },
{ id: 'product-3', category: 'books', name: 'プログラミング入門' }
];
// 各商品に対するパスを生成
return allProducts.map(product => ({
params: {
category: product.category,
productId: product.id
},
props: { product }
}));
}
const { category, productId } = Astro.params;
const { product } = Astro.props;
---
<ProductLayout title={product.name}>
<h1>{product.name}</h1>
<p>カテゴリ: {category}</p>
<p>商品ID: {productId}</p>
</ProductLayout>
現在のパスを取得して活用する
ナビゲーションの現在位置表示やパンくずリストを実装するには、現在のパスを取得する必要があります:
---
// src/components/Navigation.astro
const currentPath = Astro.url.pathname;
const navItems = [
{ path: '/', label: 'ホーム' },
{ path: '/blog/', label: 'ブログ' },
{ path: '/products/', label: '製品' },
{ path: '/about/', label: '会社情報' }
];
---
<nav>
<ul>
{navItems.map(item => (
<li class={currentPath === item.path ? 'active' : ''}>
<a href={item.path}>{item.label}</a>
</li>
))}
</ul>
</nav>
<style>
.active {
font-weight: bold;
text-decoration: underline;
}
</style>
より高度なパスユーティリティを作成するには、専用のモジュールを作成しておくと便利です:
// src/utils/paths.js
// 現在のパスがサブパスに一致するか確認(部分一致)
export function isPathMatch(currentPath, subPath) {
if (subPath === '/') {
return currentPath === '/';
}
return currentPath.startsWith(subPath);
}
// パスからセグメントを取得
export function getPathSegments(path) {
return path.split('/').filter(Boolean);
}
// パンくずリスト用のデータを生成
export function getBreadcrumbs(path, labels = {}) {
const segments = getPathSegments(path);
const breadcrumbs = [{ path: '/', label: 'ホーム' }];
let currentPath = '';
for (const segment of segments) {
currentPath += `/${segment}`;
const label = labels[currentPath] || segment;
breadcrumbs.push({ path: currentPath, label });
}
return breadcrumbs;
}
このユーティリティを使ったパンくずリストコンポーネントの例:
---
// src/components/Breadcrumbs.astro
import { getBreadcrumbs } from '../utils/paths';
const currentPath = Astro.url.pathname;
// パス名に対応するラベルのマッピング
const pathLabels = {
'/products': '製品',
'/products/electronics': '電子機器',
'/blog': 'ブログ'
};
const breadcrumbs = getBreadcrumbs(currentPath, pathLabels);
---
<nav aria-label="パンくずリスト">
<ol>
{breadcrumbs.map((crumb, index) => (
<li>
{index < breadcrumbs.length - 1 ? (
<a href={crumb.path}>{crumb.label}</a>
) : (
<span aria-current="page">{crumb.label}</span>
)}
{index < breadcrumbs.length - 1 && <span> > </span>}
</li>
))}
</ol>
</nav>
複数人開発でも迷わない命名規則と構成ルールの共有方法
大規模なプロジェクトや複数人での開発では、命名規則や構成ルールを明確にすることが重要です。これにより、チーム全体での一貫性を保ち、混乱を防ぐことができます。
命名規則の標準化
一般的に推奨される命名規則は次のとおりです:
構成ルールの標準化と共有
チーム内でディレクトリ構造や命名規則を標準化するには、以下のアプローチが有効です:
READMEファイルでの明文化
# プロジェクト構成ガイド
## ディレクトリ構造
- `src/components/`: 再利用可能なUIコンポーネント
- `ui/`: 基本的なUIコンポーネント(Button, Input等)
- `blocks/`: 複合コンポーネント(Card, Modal等)
- `sections/`: ページのセクション(Hero, Features等)
- `src/layouts/`: ページレイアウト
- `src/pages/`: ルーティング用ページ
- `src/styles/`: グローバルスタイルとスタイル変数
- `src/utils/`: ユーティリティ関数
- `public/`: 静的アセット(画像、フォント等)
## 命名規則
1. コンポーネントファイル: パスカルケース(例: `Button.astro`)
2. ユーティリティファイル: キャメルケース(例: `formatDate.js`)
3. ページファイル: ケバブケース(例: `contact-us.astro`)
## インポート順序
1. 外部ライブラリ
2. Astroコンポーネント
3. ローカルコンポーネント
4. スタイルとアセット
このREADMEをプロジェクトのルートに配置することで、すべての開発者が同じルールに従うことができます。
テンプレートファイルの提供
新しいファイルを作成する際のテンプレートを用意しておくと、一貫性が保ちやすくなります:
// component-template.astro
---
// コンポーネントプロパティ定義
export interface Props {
// プロパティをここに定義
}
// プロパティの分割代入と初期値設定
const { } = Astro.props;
---
<div class="component-name">
<slot />
</div>
<style>
.component-name {
/* スタイルを定義 */
}
</style>
ESLintとPrettierの設定
コードの一貫性を自動的に強制するには、ESLintとPrettierを設定します:
// .eslintrc.json
{
"extends": [
"plugin:astro/recommended"
],
"overrides": [
{
"files": ["*.astro"],
"parser": "astro-eslint-parser",
"rules": {
"astro/no-unused-css-selector": "error",
"astro/prefer-class-list-directive": "error"
}
}
],
"rules": {
"import/order": [
"error",
{
"groups": [
"external",
"internal",
["parent", "sibling", "index"]
],
"newlines-between": "always"
}
]
}
}
コンポーネントカタログの作成
大規模なプロジェクトでは、利用可能なコンポーネントとその使用方法を示すカタログページを作成すると便利です:
---
// src/pages/component-catalog.astro
import Layout from '../layouts/BaseLayout.astro';
// すべてのUIコンポーネントを取得
const uiComponents = import.meta.glob('../components/ui/*.astro');
const uiComponentNames = Object.keys(uiComponents).map(path => {
return path.split('/').pop().replace('.astro', '');
});
// 動的にコンポーネントをインポート
const components = await Promise.all(
uiComponentNames.map(async name => {
const path = `../components/ui/${name}.astro`;
const component = await uiComponents[path]();
return { name, Component: component.default };
})
);
---
<Layout title="コンポーネントカタログ">
<h1>UIコンポーネントカタログ</h1>
{components.map(({ name, Component }) => (
<div class="component-showcase">
<h2>{name}</h2>
<div class="component-demo">
<Component />
</div>
<details>
<summary>使用例</summary>
<pre><code>{`import ${name} from '../components/ui/${name}.astro';
<${name} />`}</code></pre>
</details>
</div>
))}
</Layout>
<style>
.component-showcase {
margin: 2rem 0;
padding: 1rem;
border: 1px solid #ddd;
border-radius: 0.5rem;
}
.component-demo {
padding: 1rem;
background-color: #f9f9f9;
border-radius: 0.25rem;
margin: 1rem 0;
}
</style>
このようなカタログページは、新しいチームメンバーのオンボーディングや、既存のコンポーネントの把握に役立ちます。
プロジェクトテンプレートの活用
頻繁に同じ構造のプロジェクトを作成する場合は、カスタムプロジェクトテンプレートを作成すると便利です。Astroでは、create-astro
コマンドで独自のテンプレートを使用できます:
# プロジェクトテンプレートからの作成
npm create astro@latest -- --template your-username/your-template-repo
独自のテンプレートレポジトリには、標準化されたディレクトリ構造、共通コンポーネント、設定ファイルなどを含めることができます。
これらの方法を組み合わせることで、複数の開発者が参加する大規模プロジェクトでも、一貫性のある構造を維持できます。また、新しいメンバーがプロジェクトに参加した際の学習コストも削減できるでしょう。
ディレクトリ構造は、単なるファイル配置のルールではなく、プロジェクトの「アーキテクチャ」を表現するものです。十分に考慮された構造は、開発効率を高め、保守性を向上させ、プロジェクトの長期的な成功に貢献します。特に、成長を続けるプロジェクトでは、初期段階からこれらの上級テクニックを適用することで、将来的な拡張がスムーズになります。

まとめ:Astroプロジェクトのディレクトリ設計ベストプラクティス
今回は、Astroプロジェクトのディレクトリ構成について、基本から応用まで詳しく解説してきました。初めてAstroを使う方にとって、ファイル構成の意味や役割を理解することは、開発をスムーズに進める上で非常に重要です。
適切なディレクトリ設計は、単なる整理整頓以上の価値があります。それは、プロジェクトの「設計図」のようなもので、開発効率、保守性、チームでの協業に大きく影響するのです。ちょうど整理された道具箱が作業をスムーズにするように、整理されたコード構造は開発作業を加速させます。
重要ポイント
特に押さえておきたい重要なポイントは以下の3つです:
- 目的に応じたディレクトリの使い分け:src/pagesはルーティング用、src/componentsは再利用可能なUI部品用、src/layoutsはページの骨格用と、それぞれの役割を理解して使い分けましょう。
- コンポーネントの適切な粒度設計:UIパーツの粒度(原子的・分子的・有機的)を意識し、再利用性と管理のしやすさのバランスを取った設計を心がけましょう。
- 拡張性を考慮した動的読み込み:import.meta.glob()などを活用した動的読み込みを導入することで、将来的な拡張にも対応できる柔軟な構造を実現できます。
初めはシンプルな構成から始めて、プロジェクトの成長に合わせてディレクトリ構造も進化させていくことをおすすめします。そして、チーム開発では、READMEなどで命名規則や構成ルールを明確に共有することで、一貫性のあるコードベースを維持できるでしょう。
Astroは比較的新しいフレームワークですが、適切なディレクトリ設計を行うことで、その真価を最大限に引き出すことができます。この記事で紹介した構成例やテクニックを参考に、あなたのプロジェクトに最適な「地図」を描いてみてください。プロジェクトが大きくなるほど、この「地図」の価値は高まっていくはずです。
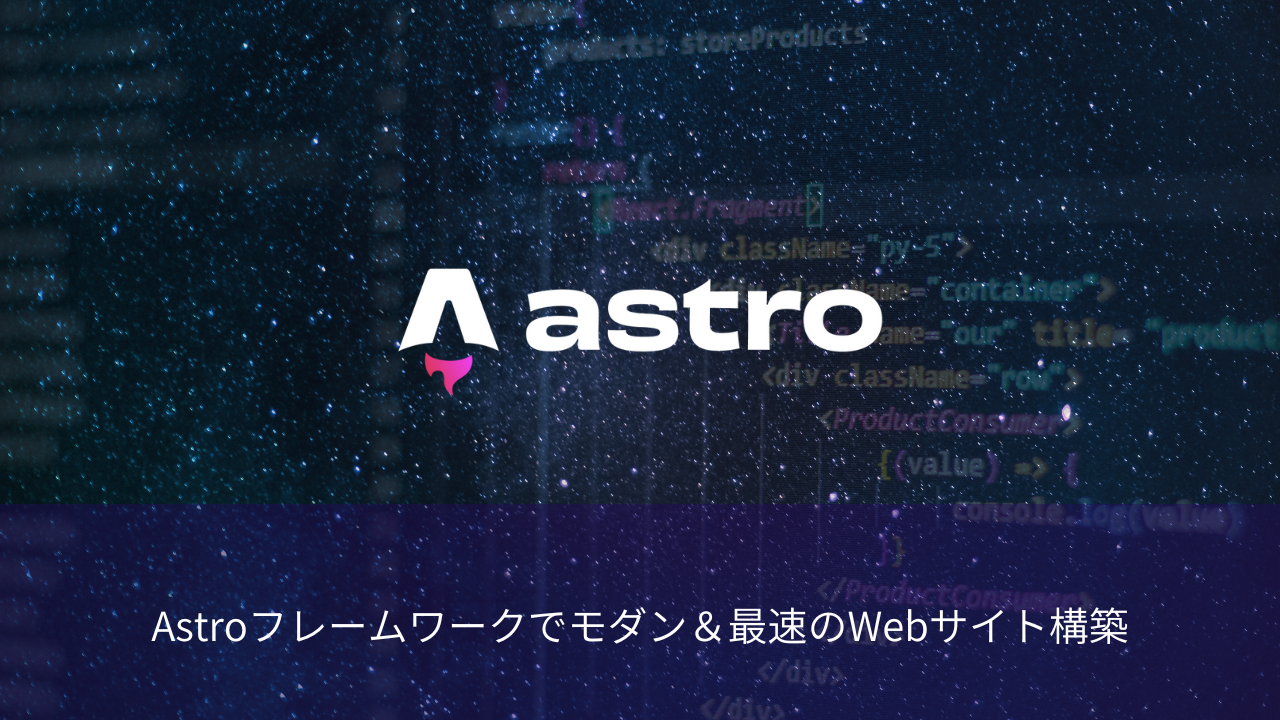
